Working with objects in JavaScript often leads to unexpected bugs. Objects in JavaScript are reference types, which makes copying them tricky. When you assign an object to a new variable, you create another reference to the same memory location. Both variables point to identical data.
Making true copies of objects becomes essential in many programming scenarios. You might need independent copies when saving application states, preserving original data, or preventing unintended side effects in your code.
Several methods exist for creating genuine copies of JavaScript objects. Each technique has specific strengths and limitations worth understanding. The right approach depends on your particular needs and object complexity.
Let’s explore practical methods showing real-world applications. By the end, you’ll know exactly which method fits your situation. Understanding these techniques will prevent common coding mistakes that waste hours of debugging.
Using Spread Operator
How the Spread Operator Works for Object Copying
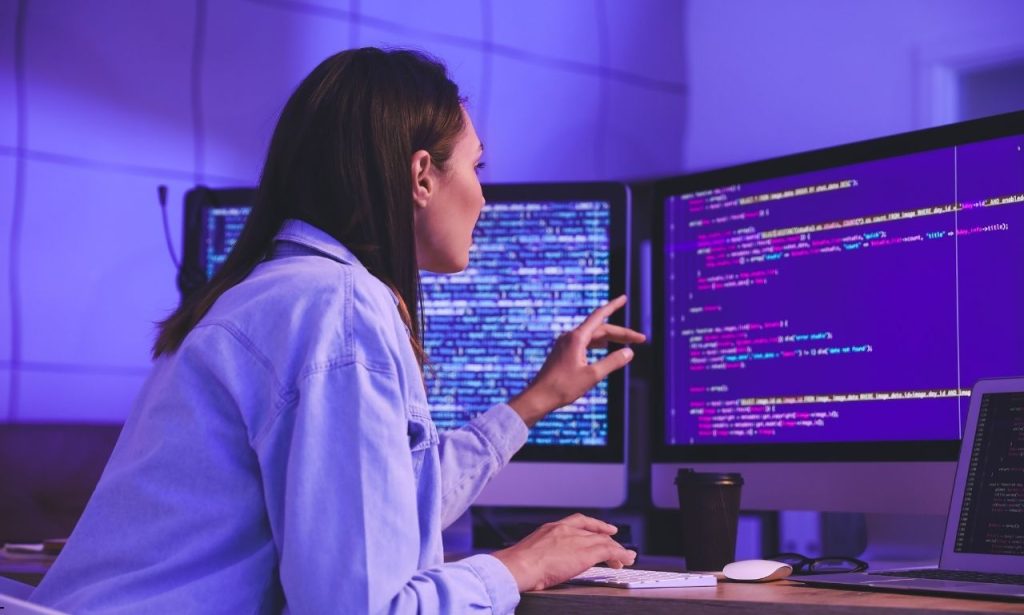
The spread operator emerged as a game-changer in ES6. It provides a quick way to copy objects with a clean syntax. This operator uses three dots before an object to extract its properties.
You can place these extracted properties within new object literals. The syntax looks remarkably straightforward compared to older methods. Most developers appreciate its readability and intuitive nature.
The spread operator creates a fresh object with the same properties. Both objects now exist independently in memory, so changes to one will not affect the other at the top level.
This method works perfectly for simple objects containing primitive values. Primitive values include strings, numbers, and booleans that get fully copied. Your new object receives complete duplicates of these values.
The operation happens quickly with minimal performance overhead. Modern JavaScript engines optimize this operator for efficient execution. It runs faster than many alternative copying methods.
Browser support for the spread operator is excellent across modern environments. All current browsers implement this feature reliably. You can use it confidently in contemporary web development projects.
Limitations of the Spread Operator
The spread operator has an important limitation to understand. It only creates shallow copies of objects. This means nested objects remain references to the original data.
Consider what happens with nested objects when copying occurs. Changing properties in nested sections affects both the original and copied versions. This happens because the nested objects maintain their reference connections.
This limitation matters when working with complex data structures. Many real-world objects contain multiple levels of nesting. The spread operator alone won’t sufficiently isolate these objects from each other.
Real applications often deal with objects containing arrays, other objects, or complex data types. These nested elements create shared references that persist after spreading. Understanding this behavior prevents unexpected bugs in your applications.
Consider this approach best for flat objects without nested structures. Those containing only primitive values work well with this method. For more complex structures, you’ll need additional techniques we’ll discuss later.
The performance benefits of spreading diminish with deeply nested objects. Each level of nesting requires additional consideration for proper isolation. Simple objects remain the ideal use case for this operator.
Using Object.assign() Method
Understanding Object.assign() for Copying
Object.assign() represents another common approach to object copying. This built-in method copies properties from source objects to a target object. It returns the modified target after the operation completes.
The method takes at least two parameters for basic operation. The first parameter becomes the target object receiving properties. Subsequent parameters serve as sources for those properties.
Multiple source objects can be provided if needed for complex operations. The method processes sources from left to right in order. Later sources can overwrite properties from earlier ones.
Many developers prefer this approach for its explicit nature. The method clearly shows its intention to copy properties between objects. Its availability across all modern browsers makes it widely compatible.
Object.assign() offers more control than the spread operator in certain scenarios. You can selectively copy properties from multiple sources into one target. This flexibility proves useful when combining data from various objects.
The method handles property enumeration automatically during the copying process. Only enumerable properties get transferred to the target object. Hidden or non-enumerable properties remain in their original locations.
The Drawbacks of Object.assign()
Object.assign() shares the same fundamental limitation as the spread operator. It only performs shallow copying of objects. Nested objects maintain their original references rather than being duplicated.
The shallow copying behavior can cause unexpected bugs in complex applications. Developers must remain aware of this limitation when working with multi-level objects. Modifying nested properties will affect all references to that object.
Performance considerations become important with large objects containing many properties. The method must iterate through each enumerable property during copying. This process takes longer with objects containing numerous fields.
Browser compatibility isn’t typically an issue with Object.assign() today. The method works reliably across all major modern browsers. Legacy support might require polyfills for very old browser versions.
The method doesn’t preserve property descriptors from the source objects. Getters and setters become regular value properties in the target. This behavior might not match your expectations in all situations.
Prototype chain properties don’t get copied during the assign operation. Only own enumerable properties transfer to the target object. Inherited properties remain with their original prototype chains.
Using JSON.parse() and JSON.stringify()
The JSON Method for Deep Copying
The combination of JSON.stringify() and JSON.parse() offers a powerful approach. This technique converts objects to JSON strings and back again. The process creates entirely new object instances at all levels.
This method works by completely serializing the object to text format. It then rebuilds the object from that text representation. The result breaks all references to the original data structure.
The JSON method requires minimal code compared to custom solutions. Most developers already understand JSON syntax, making this approach easy to comprehend. Its simplicity makes it popular for quick deep copying needs.
This technique handles nested objects and arrays correctly for most standard cases. Each level gets properly isolated from the original data structure. The copying process reaches all depths within the object hierarchy.
Performance characteristics vary depending on object size and complexity. Large objects take longer to serialize and deserialize completely. Simple objects process quickly with this method.
Memory usage temporarily doubles during the copying process. The system holds both the JSON string and rebuilt object simultaneously. This overhead matters when working with very large data structures.
Limitations of the JSON Method
Despite its advantages, the JSON technique comes with significant limitations. It can’t handle certain JavaScript features that don’t exist in JSON format. This creates potential data loss during copying.
Functions represent the most obvious limitation of this approach. JSON doesn’t support functions as values. Any methods in your objects will disappear during the serialization process.
The function loss makes the JSON method unsuitable for objects containing methods. Many complex objects in JavaScript applications include functions as essential components. Losing these methods breaks the object’s intended functionality.
Other unsupported types include undefined values, Symbols, and Map or Set objects. Date objects convert to strings rather than remaining as Date instances. RegExp objects lose their pattern and flag information during conversion.
Circular references present another major problem with this technique. Objects that reference themselves will cause errors during JSON stringification. The process can’t handle these circular structures commonly found in complex applications.
Performance can degrade significantly with deeply nested or large objects. The serialization and parsing steps become bottlenecks for complex data structures. Memory usage spikes during the intermediate string creation phase.
How to Deep Clone an Object containing a function in JavaScript?
Using Recursive Functions for Complete Cloning
Creating true deep copies of objects with functions requires custom solutions. Recursive functions provide the most reliable approach for these scenarios. They manually traverse object structures while preserving all properties.
This technique involves creating a function that scrutinizes each property type. The function makes appropriate copies based on what it finds. It calls itself recursively for nested objects or arrays.
Custom recursive functions can handle all JavaScript data types, including functions. Each type receives appropriate treatment during the copying process, and the recursion ensures that all nested structures are properly duplicated.
These functions offer complete control over the copying behavior. You can customize how different property types get handled. Special cases like circular references can be detected and managed appropriately.
The implementation complexity increases significantly compared to simpler methods. You must account for various edge cases and data types. Debugging custom recursive functions requires more effort than built-in alternatives.
Performance varies depending on object complexity and implementation efficiency. Well-optimized recursive functions can compete with built-in methods. Poorly written implementations may be slower than alternative approaches.
Memory usage patterns differ from other copying methods. The recursive approach uses stack space proportional to object depth. Very deep objects might cause stack overflow errors.
The Modern Solution: structuredClone()
The newest JavaScript environments offer a built-in solution called structuredClone(). This API provides native deep cloning capabilities without custom code. It represents the current best practice when available.
The structuredClone() function handles most complex scenarios automatically. It correctly processes nested objects, arrays, dates, and many other specialized types. This built-in approach offers better performance than manual solutions.
This function creates complete deep copies with minimal code requirements. It preserves the structure and values of complex nested objects. Most standard JavaScript objects copy correctly without special handling.
Error handling improves significantly compared to the JSON method. The function provides clear error messages for unsupported types. It fails gracefully rather than silently losing data.
Browser support for this feature continues expanding rapidly. Most modern browsers now implement structuredClone() natively, but older browsers might require fallback solutions for compatibility.
However, structuredClone() shares one limitation with the JSON method. It cannot clone functions within objects properly. Any methods in your objects will be lost during copying.
The function handles circular references correctly unlike JSON methods. Objects that reference themselves copy successfully without errors. This capability makes it suitable for complex object graphs.
Conclusion
Copying objects in JavaScript requires understanding various approaches and their trade-offs. Each method offers distinct advantages and limitations worth considering carefully. The best solution depends on your specific requirements and constraints.
For simple, flat objects, the spread operator or Object.assign() work perfectly well. These methods provide clean syntax and good performance characteristics. They’re ideal for straightforward copying needs without nested structures.
The JSON method handles deep copying of complex nested objects effectively. It creates truly independent copies at all levels of nesting. However, it fails with functions, undefined values, and circular references.
Custom recursive functions offer the most complete solution for complex objects. They can handle all data types, including functions and methods. However, the trade-off is increased code complexity and potential performance issues.
The newer structuredClone() API provides an excellent built-in option for modern environments. It combines deep copying capabilities with good performance and error handling. Function support remains its main limitation for certain use cases.
Choose your copying method carefully based on object complexity and specific needs. Understanding these techniques helps prevent unexpected bugs in your JavaScript applications. Proper object isolation leads to more predictable and maintainable code.
Testing your chosen method with representative data ensures it meets your requirements. Different copying approaches behave differently with various object types. Validation prevents surprises in production environments.
Also Read: Top 9 WordPress Themes To Use in 2025
FAQs
Shallow copying creates a new object but keeps references to nested objects. Deep copying creates completely independent copies of all nested objects and arrays.
No, the spread operator only makes shallow copies. Nested objects remain references to the original data structures.
Use a custom recursive function that specifically handles function copying. Neither JSON methods nor structuredClone() preserve functions.
No, Object.assign() only performs shallow copying. Nested objects maintain their original references.