JavaScript offers powerful tools for working with arrays. You’ve probably used loops to process array data. But there’s a better way. Map, filter, and reduce methods can make your code cleaner and more efficient. These array methods help you transform data without complex loops. They’re essential for modern JavaScript development. Today, I’ll focus on the reduce() method and its practical applications. Last month, I struggled with a project that required complex data transformations. The code was messy and hard to maintain. Then I discovered these array methods. My code became shorter and easier to understand. Let’s explore how to use map, filter, and reduce in JavaScript.
How the Reduce() Method Works
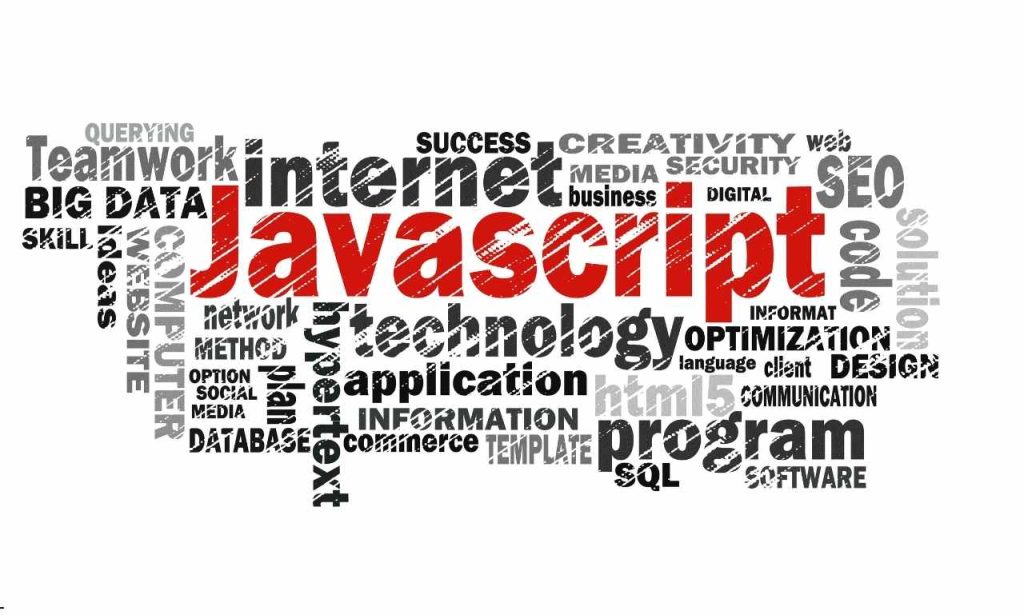
The reduce() method compresses an array into a single value. This value can be a number, string, object, or even another array. It works by applying a function to each element. The function takes an accumulator and the current element as parameters. The accumulator stores the combined result of previous operations.
Think of reduce() as a machine that processes items individually. Each item gets combined with the previous result. The machine starts with an initial value. Then it processes the first array element. The result becomes the new starting point for the next element. This continues until all elements are processed.
For example, imagine adding all the numbers in an array. You start with zero as your accumulator. The first number gets added to zero. The second number gets added to that result. This pattern continues until you’ve processed every number. The final sum represents your reduced value.
You can think of reduce() as a snowball rolling down a hill. It gathers more snow as it goes along. Each new piece of snow represents another array element. The final snowball represents your accumulated result.
The beauty of reduce() lies in its flexibility. You can use it for mathematical calculations, build complex objects from simple arrays, and create new arrays with different structures. The callback function defines what happens during each step.
When to Use the reduce() Method
The reduce() method shines in situations requiring data transformation or combination. Use it when you need to convert an array into something else. It’s perfect for calculating sums, averages, or finding maximum values. You can also build objects from arrays or flatten nested arrays.
Consider reducing when you need a single result from multiple array elements. It’s ideal for transforming arrays into objects, combining values in a custom way, and performing calculations on array elements.
For instance, calculating an average rating becomes simple with reduce(). You add all ratings together, then divide by the count. The reduce() method handles the addition part perfectly. Then you perform one division at the end.
This approach is cleaner than using traditional loops. It’s also more readable once you understand the pattern. Many developers find reduce() challenging at first. Stick with it though. The benefits are worth the learning curve.
Another common use case involves finding the maximum or minimum value. You can compare each element with the current best. The accumulator keeps track of the winning value. After processing all elements, you have your answer.
Business applications often require data aggregation. You might need to sum invoice amounts by customer. Or calculate total sales by product category. The reduce() method handles these scenarios elegantly. It transforms your raw data into meaningful insights.
How to Group Similar Items Together
Grouping is one of the most practical uses of reduce(). Let’s say you have a list of products. You want to organize them by category. The reduce() method makes this task straightforward.
Let me introduce this technique with a practical scenario. Imagine you’re building an online store. Your product array contains items from different categories. You need to display them in organized sections. Traditional loops would require multiple passes through the data. The reduce() method accomplishes this in a single pass.
The process begins with an empty object as your accumulator. For each product, you check its category. If the category doesn’t exist in your object, you create it. Then you add the product to the appropriate category group. The accumulator object grows with each iteration.
This creates an object with categories as keys. Each key contains an array of matching products. Electronics products are grouped together, and clothing items form their own group. The resulting structure makes data access much easier.
This pattern proves useful for creating dropdown menus. You can quickly list all available categories. Users can then select their preferred category. The grouped data supports efficient filtering and display.
You can adapt this technique to group by any property, group employees by department, organize events by date, or sort transactions by payment method. The flexibility makes reduce() incredibly valuable for data organization.
Restaurant apps use this technique for menu organization. They group dishes by cuisine type or dietary restrictions. Social media platforms group posts by date or author. E-commerce sites organize products by brand or price range.
How to Remove Duplicates Using the reduce() Method
Dealing with duplicate values is a common programming challenge. The reduce() method offers an elegant solution. I’ll explain how to create a unique array of values.
Let me introduce this technique for handling duplicates. User input often contains repeated values. External APIs might return duplicate records. Database queries sometimes produce redundant results. The reduce() method helps clean up this messy data.
The process starts with an empty array as your accumulator. For each element, you check if it already exists. If the element is new, you add it to your unique array. If it’s a duplicate, you skip it. This continues until all elements are processed.
The process is straightforward for simple values like numbers and strings. You check if the value exists in your accumulator array. The includes method works perfectly for this comparison. However, more complex objects require different comparison strategies.
When working with objects, you might compare specific properties. User records could be compared by email address. Product items might be unique by their ID number. The comparison logic depends on your specific requirements.
This technique proves useful when cleaning data from user inputs. Contact forms might receive duplicate submissions. Registration systems could process the same user twice. The reduce() method ensures you work with clean, unique data.
Social media applications use this to manage follower lists, preventing users from following the same person multiple times. Shopping carts use it to prevent duplicate items, and search engines use it to remove duplicate results.
Why the Initial Accumulator Value is Important
The second parameter of reduce() sets the initial accumulator value. This parameter might seem optional. But skipping it can lead to unexpected results. Always provide an initial value for clarity and consistency.
Let me explain why this matters so much. Without an initial value, reduce() uses the first array element as the accumulator. This might work for simple operations like adding numbers. But it can cause problems in more complex scenarios.
When building objects, you need an empty object as your starting point. Also, when creating arrays, you need an empty array. When calculating sums, you typically start with zero. The initial value sets the foundation for your entire operation.
This difference becomes critical when working with empty arrays. With an initial value, empty arrays return that initial value, and without an initial value, they throw an error. Many production bugs stem from this simple oversight.
Consider the implications for user-facing applications. A shopping cart might be empty, a search might return no results, or a filter might not match any items. These scenarios are common in real applications. Proper error handling starts with providing initial values.
The initial value also communicates your intentions clearly. Other developers can understand your goal immediately. They see that you’re building an object or calculating a sum. This clarity makes code maintenance much easier.
Performance considerations also favor explicit initial values. The JavaScript engine can optimize better when it knows the expected data type. This leads to faster execution and more predictable behavior.
Full reduce() Callback Parameters
The reduce() method’s callback function can accept up to four parameters. Most examples only use the first two. But knowing all four gives you more flexibility.
Let me introduce the complete parameter list. The first parameter is the accumulator that stores your combined result. The second parameter represents the current element being processed. The third parameter provides the current index position. The fourth parameter gives you access to the original array.
The accumulator parameter is the workhorse of the operation. It carries forward your results from previous iterations. Each iteration can modify this value. The final accumulator value becomes your method’s return value.
The current element parameter represents the item being processed right now. This might be a number, string, object, or any other data type. Your callback function typically uses this value in some calculation or comparison.
The index parameter helps when you need position information. You might want to process even-indexed items differently. Or skip certain positions based on business logic. The index provides this positional context.
The original array parameter proves useful when your logic depends on the whole collection. For example, you might need to calculate percentages based on the total count or reference other elements during processing. The array parameter provides this broader context.
Most times, you’ll only need the accumulator and current value. But remembering the other parameters can save you in tricky situations. They provide additional context that might be crucial for your solution.
Advanced use cases often require these extra parameters. Data analysis applications use indices for positioning. Statistical calculations reference the complete dataset. Complex transformations might need both position and context information.
Conclusion
The reduce() method is powerful in JavaScript’s array manipulation toolkit. It lets you transform arrays into any shape you need. From simple sums to complex grouping, reduce() handles it all. It might take practice to master, but the effort pays off.
Start with simple examples and gradually tackle more complex problems. Combine reduce() with map() and filter() for even more powerful transformations. These methods will make your code cleaner and more expressive, so they’re worth adding to your JavaScript toolkit.
Remember that good code communicates intent clearly. These array methods make your intentions obvious. They help other developers understand your code at a glance. So next time you reach for a traditional loop, consider whether an array method might be clearer.
Modern JavaScript development heavily relies on functional programming concepts. The reduce() method represents this paradigm perfectly. It encourages immutable data transformations. It promotes predictable, testable code patterns. These benefits extend far beyond simple array processing.
Also Read: The Best Way to Deep Copy an Object in JavaScript
FAQs
Use reduce() when transforming an array into a single value or structure. It makes your intentions clearer and often results in shorter code. For loops might be better for side effects or when you need to break early.
Map() transforms each element individually and returns a new array of the same length. Reduce() combines all elements into a single value, which can be any data type—not just an array.
Modern JavaScript engines optimize array methods well. Any performance difference is usually negligible. Focus on writing clear code first, then optimize if measurements show it’s necessary.
The standard reduce() doesn’t handle promises well. For async operations, consider using a for loop or specialized libraries. Alternatively, look into async/await with a for-of loop.